Laravel Application
Introduction to Laravel: Building a Simple Application Laravel is one of the most popular PHP frameworks for web development. It offers a clean and elegant syntax while providing robust tools to streamline tasks like routing, authentication, caching, and database management. Whether you’re building a small project or a large enterprise application, Laravel is a versatile […]
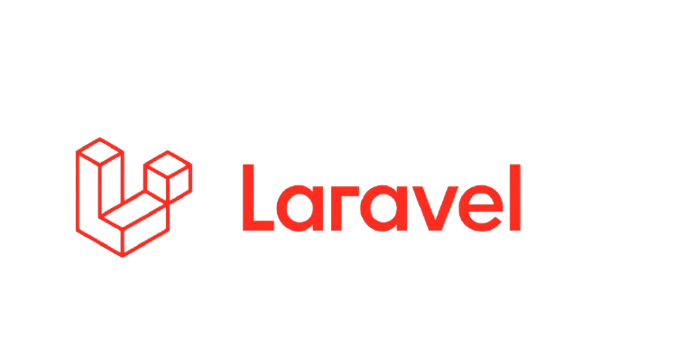
Introduction to Laravel: Building a Simple Application
Laravel is one of the most popular PHP frameworks for web development. It offers a clean and elegant syntax while providing robust tools to streamline tasks like routing, authentication, caching, and database management. Whether you’re building a small project or a large enterprise application, Laravel is a versatile and powerful framework that allows you to get the job done efficiently.
In this post, we’ll introduce Laravel and demonstrate how to create a simple Laravel application, covering the essential concepts and features that make Laravel so beloved by developers.
Why Laravel?
Before we dive into building an application, let’s explore why Laravel is so popular:
- Elegant Syntax: Laravel’s syntax is clean and expressive, making the framework easy to learn and use.
- MVC Architecture: Laravel follows the Model-View-Controller (MVC) architecture, making it easy to manage and maintain your application.
- Blade Templating: Laravel comes with a powerful templating engine called Blade, which helps you create dynamic views effortlessly.
- Eloquent ORM: Laravel’s Eloquent ORM provides a beautiful, fluent interface for interacting with your database. It simplifies database operations and makes it easier to work with models and relationships.
- Routing and Middleware: Laravel’s routing system is intuitive, and it comes with built-in middleware for handling HTTP requests.
- Security: Laravel provides robust security features like hashed passwords, SQL injection protection, and CSRF (Cross-Site Request Forgery) protection.
- Task Scheduling & Queues: Laravel allows you to schedule repetitive tasks like sending emails or generating reports. It also has a powerful queue system for deferred processing.
Setting Up Laravel
Before we build the application, make sure you have the necessary tools installed:
- PHP (preferably version 8.0 or higher)
- Composer: PHP dependency manager
- Database (MySQL, SQLite, or PostgreSQL)
1. Installing Laravel
The first step is to install Laravel. You can do this using Composer. In your terminal, run the following command:
bashCopy codecomposer create-project --prefer-dist laravel/laravel my-laravel-app
This will create a new Laravel project named my-laravel-app
.
Once the installation is complete, navigate to the project folder:
bashCopy codecd my-laravel-app
2. Setting Up the Environment
Laravel uses an .env
file to configure environment settings such as database connections, mail settings, and application keys. The .env
file should already exist in the project directory.
Open the .env
file and configure the database settings:
envCopy codeDB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=my_database
DB_USERNAME=root
DB_PASSWORD=
Make sure to replace my_database
, root
, and the password with your actual database credentials.
3. Running the Application
Once you’ve configured your environment, you can run the built-in development server to view your application. In your terminal, run:
bashCopy codephp artisan serve
This will start the server, and you can access your application by visiting http://127.0.0.1:8000
in your web browser.
Building a Simple Laravel Application
Let’s now build a simple application where users can view, create, and delete blog posts. We’ll walk through the process of creating a Post resource, which includes a database table, a model, a controller, and views.
Step 1: Create the Database Table
First, we need to create a database migration for the posts
table. In your terminal, run:
bashCopy codephp artisan make:migration create_posts_table --create=posts
This will generate a migration file in the database/migrations
folder. Open the file and define the structure of the posts
table:
phpCopy codepublic function up()
{
Schema::create('posts', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->text('content');
$table->timestamps();
});
}
Next, run the migration to create the table in the database:
bashCopy codephp artisan migrate
Step 2: Create the Post Model
Laravel’s Eloquent ORM makes it easy to interact with the database. Let’s create a Post
model:
bashCopy codephp artisan make:model Post
This will create a Post.php
file in the app/Models
directory. You can define the properties that are mass assignable in the model:
phpCopy codeclass Post extends Model
{
protected $fillable = ['title', 'content'];
}
This tells Laravel which columns can be mass-assigned when creating or updating records.
Step 3: Create the Post Controller
Now, let’s create a controller to handle our post-related logic:
bashCopy codephp artisan make:controller PostController
In the PostController.php
file, add the following methods for showing, storing, and deleting posts:
phpCopy codenamespace App\Http\Controllers;
use App\Models\Post;
use Illuminate\Http\Request;
class PostController extends Controller
{
public function index()
{
$posts = Post::all();
return view('posts.index', compact('posts'));
}
public function create()
{
return view('posts.create');
}
public function store(Request $request)
{
Post::create($request->all());
return redirect()->route('posts.index');
}
public function destroy($id)
{
Post::destroy($id);
return redirect()->route('posts.index');
}
}
Step 4: Create Routes
Next, we need to define the routes for our application in the routes/web.php
file:
phpCopy codeuse App\Http\Controllers\PostController;
Route::resource('posts', PostController::class);
The Route::resource()
method automatically generates the necessary routes for a resource controller like PostController
, including routes for viewing, creating, storing, and deleting posts.
Step 5: Create Views
Laravel uses the Blade templating engine to create dynamic views. Let’s create a view to display the posts and a form to create new posts.
resources/views/posts/index.blade.php
– List all posts:
phpCopy code<!DOCTYPE html>
<html>
<head>
<title>Laravel Blog</title>
</head>
<body>
<h1>All Posts</h1>
<a href="{{ route('posts.create') }}">Create a New Post</a>
<ul>
@foreach ($posts as $post)
<li>
<strong>{{ $post->title }}</strong>
<p>{{ $post->content }}</p>
<form action="{{ route('posts.destroy', $post->id) }}" method="POST">
@csrf
@method('DELETE')
<button type="submit">Delete</button>
</form>
</li>
@endforeach
</ul>
</body>
</html>
resources/views/posts/create.blade.php
– Form to create a new post:
phpCopy code<!DOCTYPE html>
<html>
<head>
<title>Create Post</title>
</head>
<body>
<h1>Create a New Post</h1>
<form action="{{ route('posts.store') }}" method="POST">
@csrf
<label for="title">Title</label>
<input type="text" name="title" id="title" required>
<label for="content">Content</label>
<textarea name="content" id="content" required></textarea>
<button type="submit">Save Post</button>
</form>
</body>
</html>
Conclusion
Laravel provides a powerful yet easy-to-use set of tools for building web applications. In this tutorial, we created a simple Laravel blog where users can view, create, and delete posts. By leveraging Laravel’s routing, migrations, models, controllers, and Blade templating, we were able to create a clean and maintainable web application in just a few steps.
Laravel’s built-in features like Eloquent ORM, task scheduling, and authentication make it an excellent choice for any web project, from small apps to large-scale enterprise solutions.
If you’re just starting with Laravel, explore its documentation for more in-depth information on advanced topics like authentication, authorization, and testing: Laravel Documentation.
Happy coding!